The Aethir Node Checker script will automate many useful tasks so that you can sit back, relax, and enjoy being a professional node operator 🙂 . In its current state, the script will (re)start the Aethir Checker node if the following issues occur:
- The screen session the node runs in disappeared/has been deleted
- The node CLI threw an error
- The VPS restarted itself due to an unknown reason
- A new Aethir node version has been released which is installed by the script automatically
The script was tested on Ubuntu 22.04 and will probably work with other Ubuntu versions as well. However, I don’t know if it works with other Linux distributions such as Debian, Fedora, CentOS, etc., so please be aware.
Please read everything thoroughly to understand the script and set it up properly. If you have questions, DM me (ciasis) on Discord.
Prerequisites
Before the script can be installed and used, you must already have been running an Aethir node with a working wallet in a screen session. The script doesn’t create a wallet for you.
It is important to have only one Aethir screen session running! If you’re using multiple screen sessions named Aethir, please delete them except the one that actively runs the Aethir node!
For the script, we need two packages to be installed first:
sudo apt install curl jq -y
Furthermore, the current Aethir Checker CLI file (e.g., AethirCheckerCLI-linux-1.0.2.0-eu.tar.gz) must exist inside the home directory where the script is located. The reason is that the script retrieves the name of the file on app.aethir.com and looks for it in the home directory on the VPS. If the script can’t find the file, it assumes that this “new” version of the Aethir CLI has not been downloaded yet and proceeds to download it. It then stops the current running Aethir Node session, removes it, and installs the new version.
The correct home directory should contain these items (if everything is set up properly):
- the folder that contains the node software (AethirCheckerCLI-linux-eu)
- the Aethir Node CLI file (AethirCheckerCLI-linux-1.0.2.0-eu.tar.gz)
- the script itself (AethirNodeChecker.sh)
- a log file (which will be created by the script)
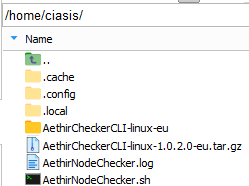
The Script
We create the script file using the text editor nano, which we call AethirNodeChecker.sh:
cd && nano AethirNodeChecker.sh
The nano editor shows us an empty file that we can populate now. It is important to replace XXX with the password of the user you logged into the VPS with. Don’t remove the quotation marks, the password must be inside them:
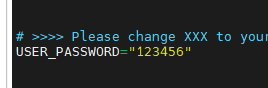
Click into the code box below to copy the script, paste it into nano, and replace XXX:
#! /bin/bash
#
# Aethir Node Checker v1.1
# by ciasis for MetaMuffin
# ------------------------
#
# Disclaimer:
# The script should be used at one's own risk.
# I don't take responsibility for any losses.
# >>>> Please change XXX to your user password and don't delete the quotes!
USER_PASSWORD="XXX"
# >>>>>>>>>>>>>>>>>>>>>>>>>>>>>><<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<
# >>>>>>>>>> > DON'T TOUCH ANYTHING FROM HERE < <<<<<<<<<<<<<<<<<<
# ---------------------------------------------------------------
# Check if another instance of this script is running:
# ---------------------------------------------------------------
pidof -o %PPID -x $0 >/dev/null && echo "ERROR: Script $0 already running" >> AethirNodeChecker.log && exit 1
# ---------------------------------------------------------------
# Save the current Aethir node working directory:
# ---------------------------------------------------------------
FOLDER_NAME=$(ls -d */ | grep 'AethirCheckerCLI')
# ---------------------------------------------------------------
# Function to start the node:
# ---------------------------------------------------------------
function StartNode()
{
if [ -f "AethirScreenLog" ]; then
rm AethirScreenLog
fi
screen -mdS "Aethir" -L -Logfile "AethirScreenLog"
screen -S "Aethir" -X stuff 'bash'`echo -ne '\015'`
sleep 1
screen -S "Aethir" -X stuff 'sudo ./AethirCheckerCLI'`echo -ne '\015'`
sleep 2
screen -S "Aethir" -X stuff $USER_PASSWORD`echo -ne '\015'`
sleep 20
screen -S "Aethir" -X stuff 'aethir license summary'`echo -ne '\015'`
}
# ---------------------------------------------------------------
# Function to extract the version number from a found FILE_NAME:
# ---------------------------------------------------------------
function extract_version()
{
local filename="$1"
if [[ "$filename" =~ ([0-9]+(\.[0-9]+)+) ]]; then
echo "${BASH_REMATCH[1]}"
else
echo ""
fi
}
# ---------------------------------------------------------------
# Restart the node if the screen session is gone for some reason:
# ---------------------------------------------------------------
if ! screen -ls | grep -q "Aethir"; then
cd $FOLDER_NAME
StartNode
cd
echo "$(date): There was no active Aethir screen session found." >> AethirNodeChecker.log
echo "$(date): The Aethir node has been restarted in a new screen session." >> AethirNodeChecker.log
fi
# ---------------------------------------------------------------
# Restart the node if the screen session throws an error:
# ---------------------------------------------------------------
cd $FOLDER_NAME
if [ -f "AethirScreenLog" ]; then
lastline=$(tail -n 1 AethirScreenLog)
if [[ $lastline =~ "error" ]]; then
cd
echo "$(date): The Aethir node experienced an error and will be restarted now." >> AethirNodeChecker.log
ID=$(screen -ls | awk '/[0-9]+\.(.*Aethir.*)/ {print $1}' | cut -d'.' -f1)
screen -XS $ID quit
cd $FOLDER_NAME
StartNode
cd
echo "$(date): The Aethir node has been restarted in a new screen session." >> AethirNodeChecker.log
fi
else
cd
echo "$(date): There was no AethirScreenLog file found." >> AethirNodeChecker.log
fi
# ---------------------------------------------------------------
# Download the lastest node version and start the node:
# ---------------------------------------------------------------
SITE_URL="https://app.aethir.com/console-api/client/download-conf"
DOWNLOAD_URL=$(curl -s $SITE_URL | jq -r '.data[] | select(.name | contains("Linux")) | .url')
# Check the DOWNLOAD_URL can be retrieved:
if [[ -z "$DOWNLOAD_URL" ]]; then
echo "$(date): Failed to retrieve the download URL for the Linux CLI." >> AethirNodeChecker.log
exit 1
fi
FILE_NAME=$(basename $DOWNLOAD_URL)
# Extract the version number from FILE_NAME and check, if a version number was found, and if not, exit:
TARGET_VERSION=$(extract_version "$FILE_NAME")
if [[ -z "$TARGET_VERSION" ]]; then
echo "$(date): The version number in the file name of the Aethir node to be downloaded is missing, so I can't continue to download the new node version." >> AethirNodeChecker.log
exit 1
fi
# Flag to track if the version is found:
found_flag=0
# Search for files containing "AethirCheckerCLI" in the current directory and compare their version numbers:
cd
for file in *AethirCheckerCLI*; do
if [[ -f "$file" ]]; then
FILE_VERSION=$(extract_version "$file")
if [[ "$FILE_VERSION" == "$TARGET_VERSION" ]]; then
found_flag=1
break
fi
fi
done
# If no matching version number was found, the new Aethir node will be downloaded:
if [[ $found_flag -eq 0 ]]; then
curl -O $DOWNLOAD_URL
echo "$(date): Downloaded the new Aethir node version named $FILE_NAME." >> AethirNodeChecker.log
ID=$(screen -ls | awk '/[0-9]+\.(.*Aethir.*)/ {print $1}' | cut -d'.' -f1)
screen -XS $ID quit
echo $USER_PASSWORD | sudo -S rm -rf $FOLDER_NAME
tar -xzf $FILE_NAME
NEW_FOLDER_NAME=$(ls -d */ | grep 'AethirCheckerCLI')
cd $NEW_FOLDER_NAME
echo $USER_PASSWORD | sudo -S bash install.sh
StartNode
cd
echo "$(date): The new Aethir node has been started." >> AethirNodeChecker.log
fi
Hit STRG (CTRL) + X on your keyboard, confirm the question of saving the modified buffer with y (yes) and hit Enter. Good, the script is created. Next, we need to make it executable, because otherwise, the system daemon cron can’t run it. Let’s give execute permissions to our script:
chmod +x AethirNodeChecker.sh
Important to know:
- The script always creates a screen session called “Aethir”
- When the script creates a new screen session, it also creates a log file called AethirScreenLog inside the node folder. The content of the log file is the same as what you see when you reattach to the Aethir screen session, which means, it’s not necessary to reattach anymore but to look into the log file which mirrors everything happening in the screen session. The content can be viewed by moving into the node folder using cd AethirCheckerCLI-linux-eu and then cat AethirScreenLog.
- There is always one folder with the node software, in which the active node runs, not multiple folders (the folder is called something like AethirCheckerCLI-linux-eu)
- If a new Aethir node version is downloaded, the file is not deleted, only its content is extracted (the new node folder)
- The extracted content is a folder that replaces the current Aethir node folder (the old node folder will be deleted)
The Cron Job
We’re done with the script part. The next chapter is about creating the cron job to run the script automatically. We need a command that the cron daemon runs for us to do this. This command is structured as follows:
Time-frequency | Command to execute | Output
There are several websites out there that help you generate a cron job, especially the time-frequency part. One is Crontab-Generator.org, another one is crontab guru. Here, you can be flexible regarding the frequency, and how often the script should be run. Regarding the command to execute, this part contains the path to the script. The output can be written to a file, sent via e-mail, or just written to the trashcan. We want the latter. But before we look at the command, we must create a crontab file to put our command into, so the daemon can read and run it. To create a crontab file or open it, we use this command:
crontab -e
If you haven’t executed this command before, the system will ask you which text editor you prefer. We’re going to use nano, so hit the number 1 key and then Enter:
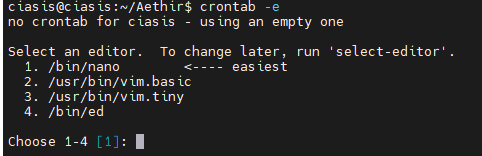
You’re presented with a lot of text. Ignore that stuff. Move the cursor down to the end below the last written line. We’re going to use the following command for the cron job to run the script every minute:
*/1 * * * * /home/ciasis/AethirNodeChecker.sh
Pay attention here: you need to edit the path and change the user name to your user or just replace everything so that the path ends with the script file name. Then paste the command into the crontab file. Now it looks like this:

Save the file by STRG (CTRL) + X, hit y, and Enter. That’s it, the cron job is active. You can list all active jobs for your user by executing this command:
crontab -l
To monitor and check for past cron job runs (and to make sure our job is run correctly), we can look at the system logs by using this command:
sudo grep CRON /var/log/syslog
If you see stuff like this, then it’s good:

You can also have a look at the log file the script is writing to by using this command:
cat AethirNodeChecker.log
Feel free to send me any feedback if something doesn’t work.